

However, for real applications, you should use Redux Toolkit to write your Redux logic. Redux Toolkit is beneficial to all Redux users regardless of skill level or experience. The "hand-written" Redux logic you've written so far in this tutorial is actual working code, but you shouldn't write Redux logic by hand - we've covered those approaches in this tutorial so that you understand how Redux works. Redux Toolkit makes it easier to write good Redux applications and speeds up development, by baking in our recommended best practices, providing good default behaviors, catching mistakes, and allowing you to write simpler code. Redux Toolkit builds in our suggested best practices, simplifies most Redux tasks, prevents common mistakes, and makes it easier to write Redux applications.īecause of this, Redux Toolkit is the standard way to write Redux application logic. Redux Toolkit contains packages and functions that we think are essential for building a Redux app. That's why the Redux team created Redux Toolkit: our official, opinionated, "batteries included" toolset for efficient Redux development. Finally, many times users aren't sure what "the right way" is to write Redux logic. In addition, the process for setting up a Redux store takes several steps, and we've had to come up with our own logic for things like dispatching "loading" actions in thunks or processing normalized data.
#Reduxjs toolkit code
There's good reasons why these patterns exist, but writing that code "by hand" can be difficult. Normalized state makes it easier to look up items by IDsĪs you've seen, many aspects of Redux involve writing some code that can be verbose, such as immutable updates, action types and action creators, and normalizing state.Request status should be tracked with loading state enum values.Memoized selectors optimize calculating transformed data.Action creators encapsulate preparing action objects and thunks.

Redux "thunk" functions are the standard way to write basic async logic.Middleware add an extra step to the Redux data flow, enabling async logic.Redux middleware allow writing logic that has side effects.wraps your app and lets components access the store.useDispatch lets components dispatch actions.useSelector reads values from Redux state and subscribes to updates.React-Redux provides APIs to let React components talk to Redux stores.Redux is separate from any UI, but frequently used with React.The Redux DevTools extension lets you see how your state changes over time.Stores can be customized using "enhancers" and "middleware".The createStore API creates a Redux store with a root reducer function.Reducers must follow rules like "immutable updates" and "no side effects".Reducers take current state and an action, and calculate a new state.Actions are objects that describe "what happened" events in an app.
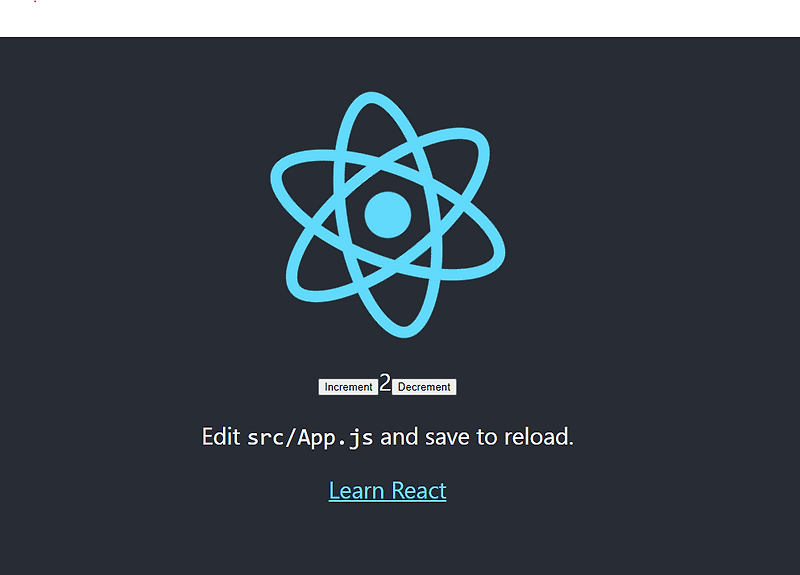
How Redux uses a "one-way data flow" pattern.what Redux is, when/why to use it, and the basic pieces of a Redux app.Nevertheless I think this is a great step forward in simplifying Redux, and I’m interested to see where things goes in the future. I understand there may be some good reasons behind it, but I think it could confuse developers new to Redux into thinking it was optional, when I think it really should become compulsory and the new standard. It’s also interesting that the toolkit lives in its own separate library, instead of being part of the main react-redux library. I could see this getting confusing and hard to manage, especially for developers who are new to the codebase. New Redux with hooks like useSelector().Unfortunately, for developers working on older and larger codebases, you could end up having to wrap your head around multiple ways of writing Redux:
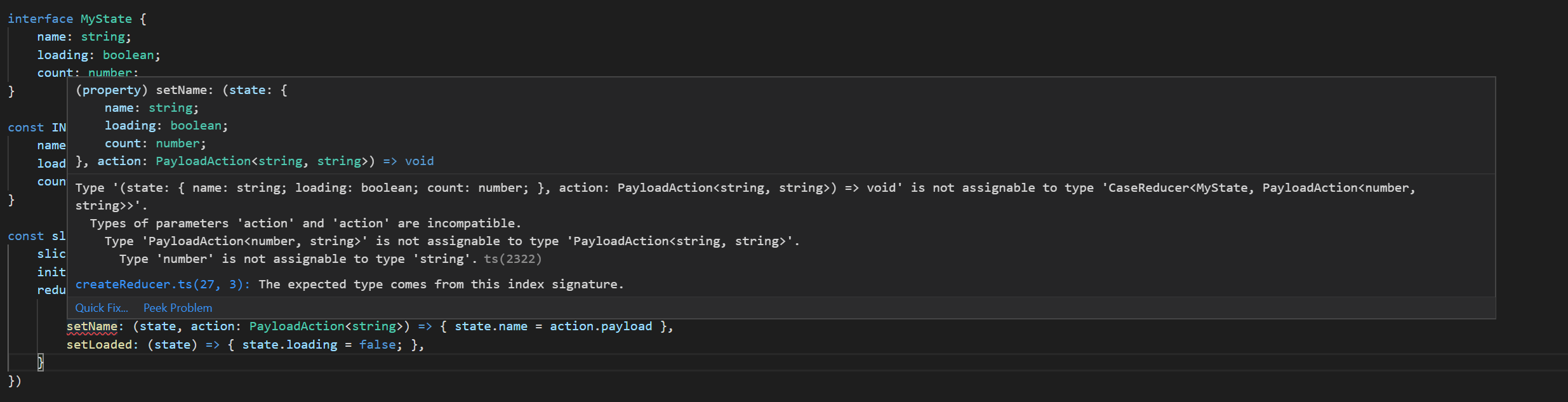
If you’re a developer working on a new codebase, I’d recommend jumping straight into using this toolkit. By default it also provides useful tools that many codebases will need, like createSelector and redux-thunk. Redux Toolkit is a great library for simplifying the Redux code you write. RTK Query is provided as an optional addon within the reduxjs/toolkit package. Since this middleware is so useful, it has been included by default when you set up your store using configureStore(). Now our component code no longer has to know whether it needs to pass in dispatch as an argument or not, and can always just wrap actions in the dispatch function!
